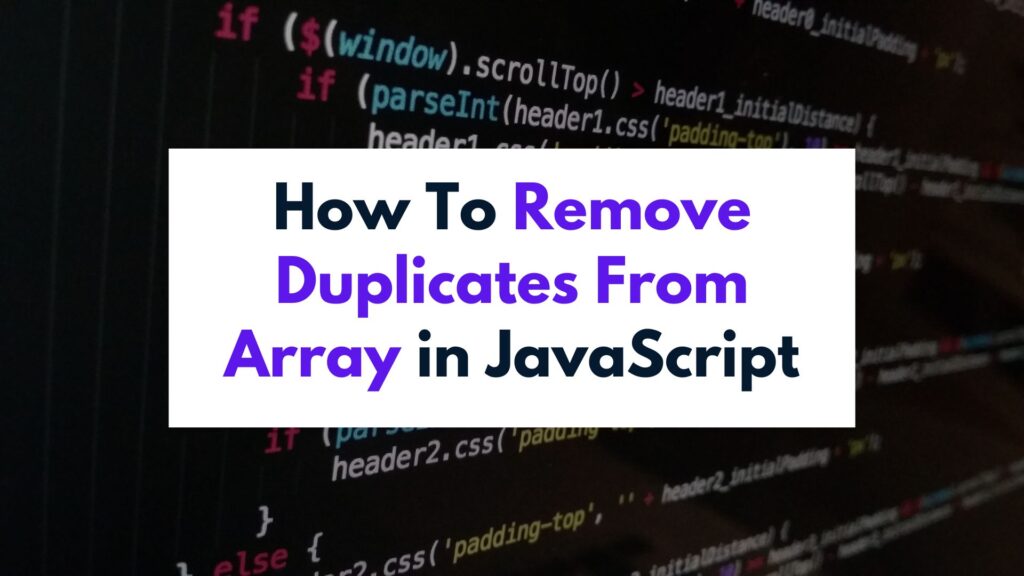
In JavaScript, dealing with arrays is a common task for developers. Oftentimes, arrays may contain duplicate elements, and it becomes essential to eliminate these duplicates to ensure accurate data representation and efficient processing. In this article, we will explore various methods to remove duplicates from arrays in JavaScript, accompanied by examples and in-depth explanations.
4 Methods To Remove Duplicates From Array in JavaScript
Method 1) Using Set:
One of the most straightforward and modern ways to remove duplicates is by utilizing the Set
object. A Set
automatically removes duplicate values, ensuring that only unique elements remain in the array.
const arrayWithDuplicates = [1, 2, 3, 4, 1, 2, 5]; const uniqueArray = [...new Set(arrayWithDuplicates)]; console.log(uniqueArray);
This method works well for arrays of primitive data types like numbers and strings. However, for arrays of objects, the Set
method may not be sufficient.
Method 2) Filter and indexOf:
Another approach involves using the filter
method in combination with the indexOf
method to create a new array with only the first occurrence of each element.
const arrayWithDuplicates = [1, 2, 3, 4, 1, 2, 5]; const uniqueArray = arrayWithDuplicates.filter((value, index, self) => { return self.indexOf(value) === index; }); console.log(uniqueArray);
This method is suitable for arrays with both primitive data types and objects. However, it may not be the most performant solution for large arrays.
Method 3) Using reduce:
The reduce method can also be employed to eliminate duplicates by building a new array while checking for the existence of each element.
const arrayWithDuplicates = [1, 2, 3, 4, 1, 2, 5]; const uniqueArray = arrayWithDuplicates.reduce((accumulator, currentValue) => { if (!accumulator.includes(currentValue)) { accumulator.push(currentValue); } return accumulator; }, []); console.log(uniqueArray);
While this method is effective, it may not be as concise as some of the other approaches.
Method 4) Using ES6 Set with Arrays of Objects:
When working with arrays of objects, using Set
may not directly eliminate duplicates. In such cases, a combination of map
and JSON.stringify
can be used.
const arrayOfObjectsWithDuplicates = [{id: 1}, {id: 2}, {id: 1}, {id: 3}]; const uniqueArrayOfObjects = Array.from(new Set(arrayOfObjectsWithDuplicates.map(JSON.stringify))).map(JSON.parse); console.log(uniqueArrayOfObjects);
This approach converts each object to a JSON string, removes duplicates, and then converts them back to objects.
Conclusion
Removing duplicates from arrays in JavaScript is a common task, and there are multiple ways to achieve this goal. The choice of method depends on the specific requirements of your project and the nature of the data in the array. Whether you opt for the simplicity of the Set
method or the flexibility of the filter
and indexOf
combination, understanding these techniques equips you with the tools to efficiently handle arrays in your JavaScript projects.