In JavaScript, dealing with strings is a common task for developers. Oftentimes, you may encounter scenarios where you need to remove numbers from a string. Whether you’re working with user inputs, parsing data, or manipulating strings, knowing how to remove numbers from a string is a valuable skill. In this article, we’ll explore various techniques and methods to achieve this in JavaScript.
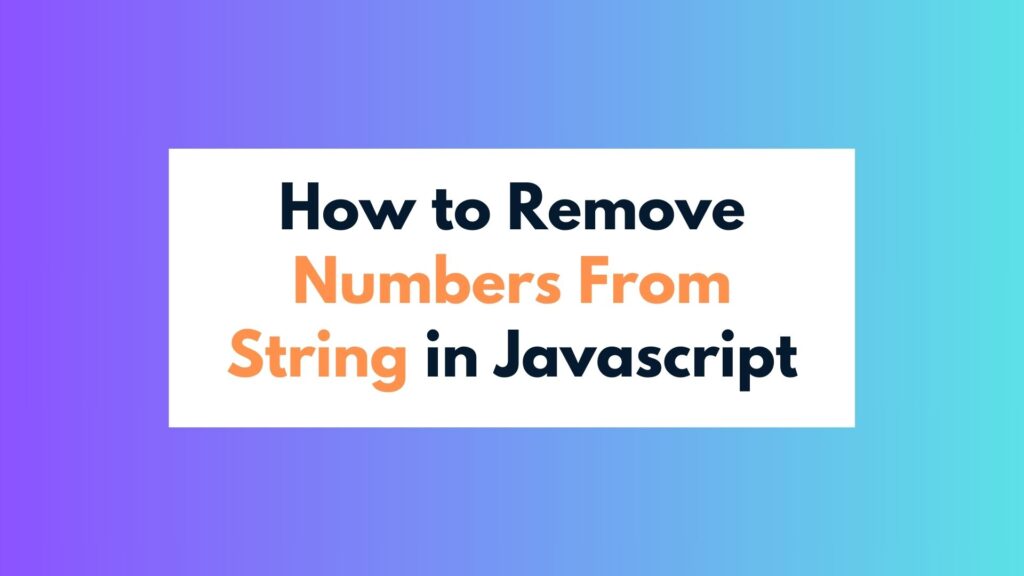
Table of Contents
Method 1: Using Regular Expressions
One of the most powerful and flexible ways to remove numbers from a string is by leveraging regular expressions.
const stringWithNumbers = "Hello123World456"; const stringWithoutNumbers = stringWithNumbers.replace(/\d+/g, ''); console.log(stringWithoutNumbers);
Explanation:
/\d+/g
: This regular expression targets one or more digits globally (the ‘g’ flag).replace()
: Thereplace
method is used to replace the matched digits with an empty string.
Method 2: Using a Loop and isNaN()
If you prefer a more procedural approach, you can use a loop along with the isNaN()
function.
const stringWithNumbers = "Hello123World456"; let stringWithoutNumbers = ''; for (let char of stringWithNumbers) { if (isNaN(char)) { stringWithoutNumbers += char; } } console.log(stringWithoutNumbers);
Explanation:
- The
isNaN()
function checks if a value is NaN (Not-a-Number). - The loop iterates through each character in the string, and if it’s not a number, it’s appended to the result string.
Method 3: Splitting and Filtering
Another technique involves splitting the string into an array and filtering out the numeric elements.
const stringWithNumbers = "Hello123World456"; const stringWithoutNumbers = stringWithNumbers.split('').filter(char => isNaN(char)).join(''); console.log(stringWithoutNumbers);
Explanation:
split('')
: Splits the string into an array of characters.filter(char => isNaN(char))
: Filters out the numeric characters using theisNaN()
function.join('')
: Joins the filtered array back into a string.
Method 4: Using Regular Expressions with Replace Callback
For more advanced scenarios, you can use a callback function with the replace()
method.
const stringWithNumbers = "Hello123World456"; const stringWithoutNumbers = stringWithNumbers.replace(/\d+/g, match => { // Custom logic to replace each match return ''; }); console.log(stringWithoutNumbers);
Explanation:
- The callback function allows for custom logic for each match found by the regular expression.
Conclusion: Removing numbers from a string in JavaScript can be accomplished using various methods, each with its own advantages. Whether you choose regular expressions, loops, or a combination of methods, understanding these techniques equips you with the skills needed to manipulate strings effectively. Experiment with these examples, and choose the approach that best fits your specific use case.