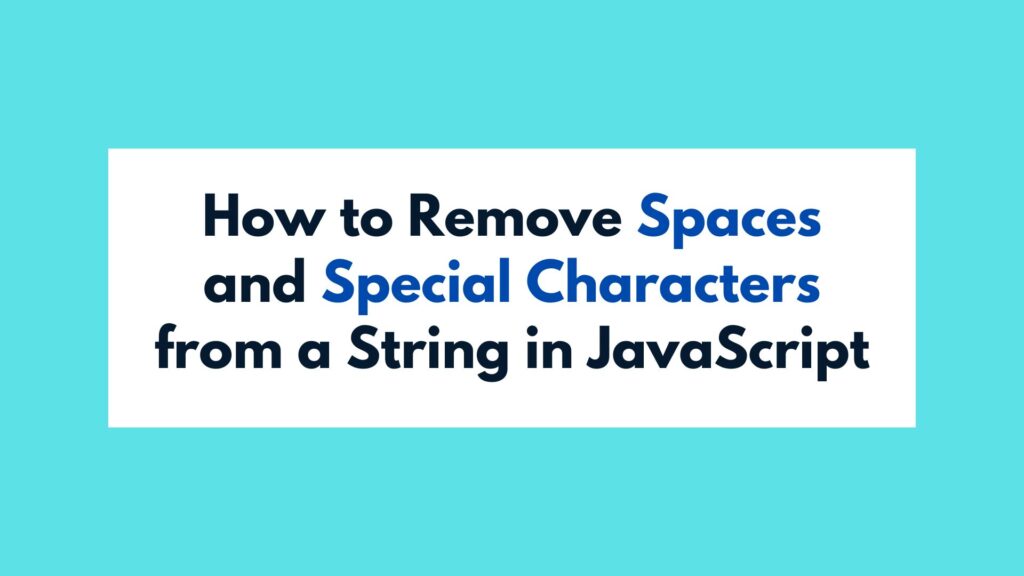
Cleaning strings in JavaScript is a common task for developers. Unwanted spaces or special characters in user input can break functionality or cause errors. Whether you’re sanitizing form inputs, creating clean usernames, or formatting URLs, removing these characters ensures your app runs smoothly. This article covers reliable methods to clean strings, with examples and tips.
Table of Contents
Quick Answer Summary
How to remove spaces and special characters in JavaScript?
Use the replace()
method with a regular expression (regex) to remove spaces and special characters. This approach is fast and flexible for most use cases.
let str = "Hello! How are you?"; let cleanStr = str.replace(/[^a-zA-Z0-9]/g, ''); console.log(cleanStr); // Output: HelloHowareyou
Why Clean Strings in JavaScript?
Unwanted spaces or special characters can cause issues like:
- Validation Errors: Extra spaces in form inputs can fail checks.
- Security Risks: Special characters might allow injection attacks if not sanitized.
- Formatting Issues: URLs or database queries may break with invalid characters.
Cleaning strings is essential for:
- Usernames (e.g., ensuring “john@doe!” becomes “john_doe”).
- Form data (e.g., removing spaces from phone numbers).
- URL slugs (e.g., converting “My Blog Post!” to “my-blog-post”).
Method 1: Using Regex
Regular expressions (regex) are powerful for string cleaning. The replace()
method with regex patterns can target specific characters.
Remove All Special Characters
This pattern removes everything except letters, numbers, and spaces:
let str = "Hello! How@are#you?"; let cleanStr = str.replace(/[^\w\s]/gi, ''); console.log(cleanStr); // Output: Hello Howareyou
- Pattern Explained:
\w
: Matches letters, numbers, and underscores.\s
: Matches spaces.[^\w\s]
: Matches anything not a letter, number, underscore, or space.g
: Applies globally.i
: Ignores case.
Remove Only Spaces
To remove only spaces, target the space character:
let str = "Hello World!"; let noSpaces = str.replace(/\s/g, ''); console.log(noSpaces); // Output: HelloWorld!
- Pattern Explained:
\s
: Matches any whitespace (spaces, tabs, etc.).g
: Removes all matches.
Allow Only Alphanumeric
To keep only letters and numbers:
let str = "Hello@World! 2023"; let alphanumeric = str.replace(/[^a-z0-9]/gi, ''); console.log(alphanumeric); // Output: HelloWorld2023
- Pattern Explained:
[^a-z0-9]
: Matches anything not a letter or number.g
: Global replacement.i
: Case-insensitive.
Method 2: Using Built-in JavaScript String Functions
For simpler cases, JavaScript’s built-in string methods like split()
, filter()
, join()
, or trim()
work well. These are easier to read but less flexible than regex.
Remove Spaces with trim()
and split()/join()
trim()
: Removes leading/trailing spaces.split()/join()
: Splits a string into an array and joins it back.
let str = " Hello World! "; let noSpaces = str.trim().split(' ').join(''); console.log(noSpaces); // Output: HelloWorld!
Remove Special Characters with filter()
Convert the string to an array, filter out unwanted characters, and join:
let str = "Hello@World!"; let cleanStr = str .split('') .filter(char => /[a-zA-Z0-9]/.test(char)) .join(''); console.log(cleanStr); // Output: HelloWorld
When to Use String Methods
- Use for simple tasks (e.g., removing only spaces).
- Better for readability if regex feels complex.
- Avoid for complex patterns (regex is more efficient).
Complete Working Example
Here’s a full example that cleans a user input string, removing spaces and special characters:
function cleanString(input) { // Remove special characters and spaces, keep alphanumeric return input.replace(/[^a-zA-Z0-9]/g, ''); } // Example usage let userInput = "Hello! How are you? 2023@"; let cleaned = cleanString(userInput); console.log(cleaned); // Output: HelloHowareyou2023 // Test with different inputs let inputs = [ "John@Doe 123!", "My#Blog Post!", " Space Test! " ]; inputs.forEach(input => { console.log(`Original: ${input} → Cleaned: ${cleanString(input)}`); });
Output:
HelloHowareyou2023 Original: John@Doe 123! → Cleaned: JohnDoe123 Original: My#Blog Post! → Cleaned: MyBlogPost Original: Space Test! → Cleaned: SpaceTest
This code is reusable and works for form inputs, usernames, or URLs.
FAQs
How do I remove only spaces from a string in JS?
Use str.replace(/\s/g, '')
to remove all spaces. Alternatively, use str.split(' ').join('')
for readability.
What is the best way to remove special characters from a string?
The regex method str.replace(/[^a-zA-Z0-9]/g, '')
is the most flexible and efficient for removing special characters while keeping alphanumeric ones.
Is regex the most efficient way to clean strings?
Regex is often the fastest for complex patterns. For simple tasks like removing spaces, string methods like trim()
or split()/join()
can be sufficient and more readable.
Conclusion
Cleaning strings in JavaScript is straightforward with regex or string methods. Regex (str.replace(/[^a-zA-Z0-9]/g, '')
) is the go-to for most cases due to its flexibility and speed. String methods like trim()
or split()/join()
are great for simpler tasks. Test your code with varied inputs to ensure reliability.