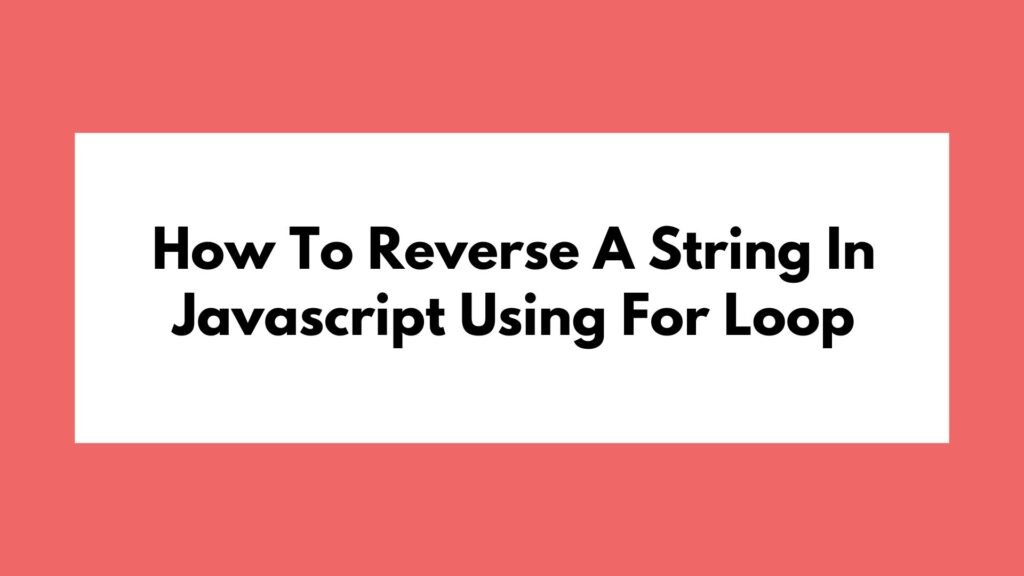
In JavaScript, reversing a string is a common task that can be achieved using various methods. One of the simplest and most fundamental ways to reverse a string is by utilizing a for loop. In this article, we will explore how to reverse a string in JavaScript efficiently using a for loop.
Understanding the Process
Before delving into the coding aspect, it is essential to grasp the logic behind reversing a string using a for loop. The basic idea is to iterate through the characters of the input string in reverse order and concatenate them to form the reversed string.
To Reverse A String In Javascript Using For Loop, We Can Follow These Steps:
Let’s break down the process into simple steps:
1. Initialize Variables
To begin, we need to declare two variables: one for storing the original string and another for holding the reversed string.
let str = "Hello, World!"; let reversedStr = "";
2. Iterate Using a For Loop
Next, we will use a for loop to iterate through the characters of the original string in reverse order.
for (let i = str.length - 1; i >= 0; i--) { reversedStr += str[i]; }
In this for loop:
i
starts at the last index of the string (str.length - 1
).- The loop continues while
i
is greater than or equal to 0. - We concatenate each character from the original string to
reversedStr
starting from the last character.
3. Display the Reversed String
Finally, we can output the reversed string to see the result.
console.log(reversedStr); // Output: "!dlroW ,olleH"
Full Code Example
Putting it all together, here is the complete JavaScript code for reversing a string using a for loop:
let str = "Hello, World!"; let reversedStr = ""; for (let i = str.length - 1; i >= 0; i--) { reversedStr += str[i]; } console.log(reversedStr); // Output: "!dlroW ,olleH"
Conclusion
In conclusion, reversing a string in JavaScript using a for loop is a straightforward process that involves iterating through the characters of the original string in reverse order. By following the steps outlined in this article, you can effectively reverse any given string with ease.