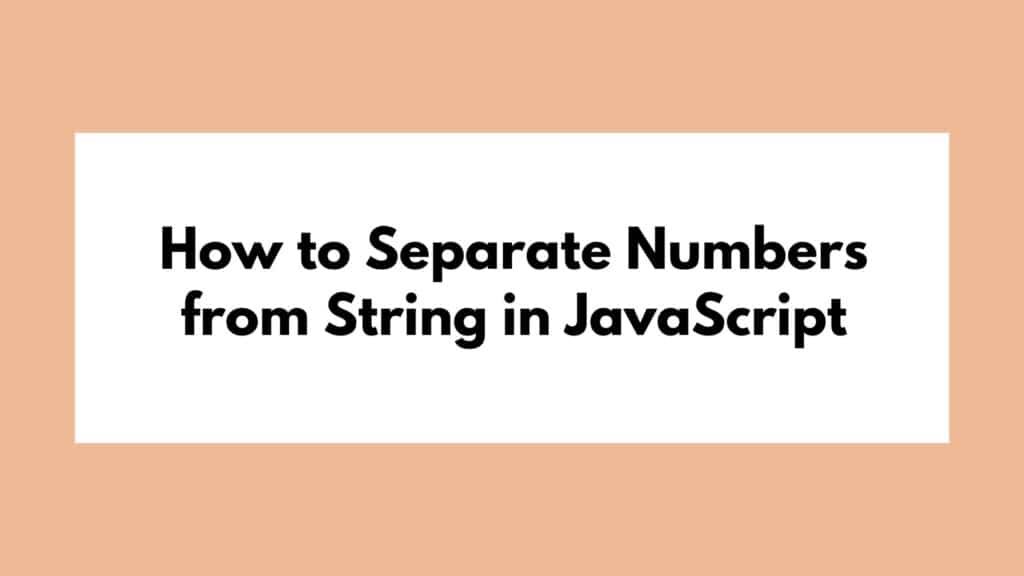
When working with JavaScript, separating numbers from a string is a common task that can be crucial for various data processing and validation scenarios. In this article, we will explore an efficient method to achieve this separation.
Table of Contents
Understanding the Problem
Before diving into the code implementation, it’s essential to grasp the challenge at hand. We aim to extract only numerical values from a string that contains a mix of numbers and non-numeric characters.
Using Regular Expressions
One of the most effective ways to separate numbers from a string in JavaScript is by leveraging regular expressions. Regular expressions offer a powerful tool for matching patterns within strings.
Step 1: Code Implementation
Let’s look at an example code snippet using regular expressions to extract numbers from a string:
const inputString = 'hello123world456'; const numbersArray = inputString.match(/\d+/g).map(Number); console.log(numbersArray);
Step 2: Code Explanation
inputString.match(/\d+/g)
: Matches one or more digits in the input string.map(Number)
: Converts each matched sequence into a number using theNumber
function.
Additional Example
Consider another example to reinforce the concept:
const text = 'apple123banana456carrot'; const numbers = text.match(/\d+/g).map(Number); console.log(numbers);
Conclusion
Separating numbers from a string in JavaScript can be efficiently accomplished using regular expressions. By following the step-by-step guide and examples outlined in this article, you can effectively extract numerical values from strings in your JavaScript projects.