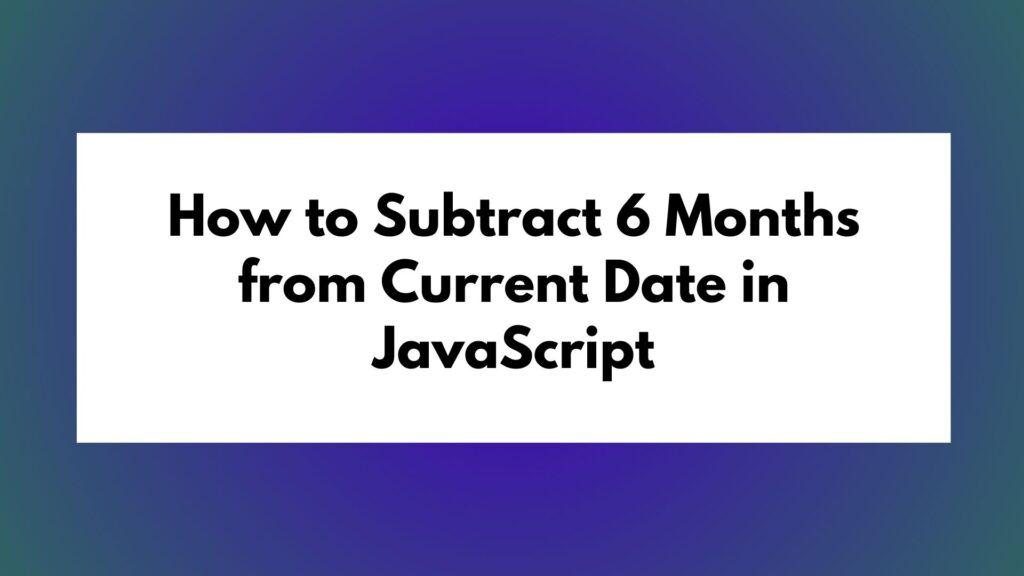
In the world of programming, manipulating dates is a common task that developers encounter. One particular operation that may come up is subtracting a specific duration from the current date. In this article, we will focus on how to subtract 6 months from the current date using JavaScript. We will explore different methods to achieve this with clear and concise explanations.
Methods to Subtract 6 Months from Current Date in JavaScript
Method 1: Using JavaScript Date Object
The first method involves utilizing the built-in Date
object in JavaScript. Here’s a step-by-step breakdown of how you can subtract 6 months from the current date using this method:
- Get the current date:
const currentDate = new Date();
- Subtract 6 months:
currentDate.setMonth(currentDate.getMonth() - 6);
- Retrieve the updated date:
const updatedDate = currentDate.toDateString();
Full Code Snippet:
// Method 1: Using JavaScript Date Object const currentDate = new Date(); currentDate.setMonth(currentDate.getMonth() - 6); const updatedDate = currentDate.toDateString(); console.log(updatedDate);
Method 2: Using External Libraries
Another approach is to leverage external libraries like moment.js
for date manipulation. Below is a detailed guide on how to achieve the same result using moment.js
:
- Install
moment.js
library:
npm install moment
- Import
moment
in your JavaScript file:
const moment = require('moment');
- Subtract 6 months from the current date:
const currentDate = moment(); const updatedDate = currentDate.subtract(6, 'months').format('MMMM Do YYYY');
Full Code Snippet:
// Method 2: Using External Libraries (moment.js) const moment = require('moment'); const currentDate = moment(); const updatedDate = currentDate.subtract(6, 'months').format('MMMM Do YYYY'); console.log(updatedDate);
Conclusion
In this article, we have explored two methods for subtracting 6 months from the current date in JavaScript. By following the provided steps and using the full code snippets, you can efficiently perform this operation in your projects. Remember to choose the method that best suits your requirements and coding style. Happy coding!