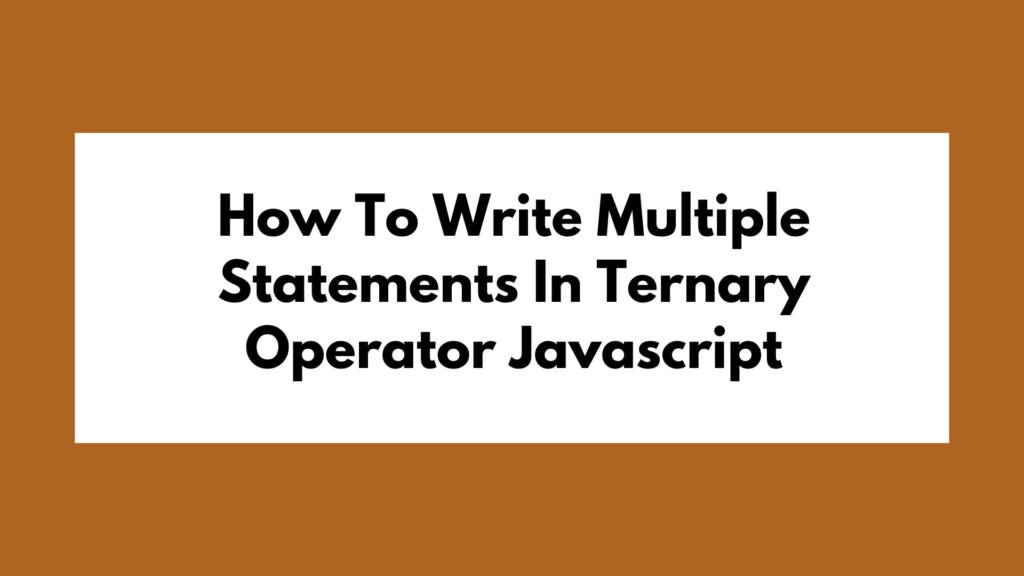
In the realm of JavaScript programming, the ternary operator provides a concise way to write conditional statements. However, many developers are unaware of its potential for handling multiple statements effectively. In this article, we will delve into the intricacies of writing multiple statements within a ternary operator, offering step-by-step guidance and detailed examples.
Table of Contents
Understanding the Ternary Operator
Before we explore the advanced usage of the ternary operator, let’s revisit its basic syntax:
condition ? expression1 : expression2;
Here, condition
is evaluated, and if it resolves to true
, expression1
is executed; otherwise, expression2
is executed.
Writing Multiple Statements
To incorporate multiple statements within a ternary operator, we can leverage JavaScript’s ability to use block statements with curly braces. By encapsulating the statements within curly braces, we can execute a sequence of actions based on the condition.
condition ? (statement1, statement2, statement3) : (statement4, statement5);
In the above example, if condition
is true
, statement1
, statement2
, and statement3
will be executed in sequence. Conversely, if condition
is false
, statement4
and statement5
will be executed.
Step-by-Step Explanation
Let’s break down the process of writing multiple statements in a ternary operator:
- Evaluate the Condition: Begin by evaluating the condition specified before the
?
. - Use Curly Braces: Enclose the multiple statements you wish to execute within curly braces.
- Separate Statements with Commas: Separate each statement within the curly braces using commas.
- Execute Based on Condition: Depending on whether the condition is
true
orfalse
, the corresponding block of statements will be executed.
Example Code Snippets
Example 1: Basic Implementation
const result = (condition) ? ( statement1(), statement2() ) : ( statement3(), statement4() );
In this example, statement1
and statement2
will be executed if the condition
is true
; otherwise, statement3
and statement4
will be executed.
Example 2: Handling Variables
const message = (isSuccess) ? ( successMessage = "Operation successful", showSuccessPopup() ) : ( errorMessage = "Operation failed", showErrorPopup() );
Here, based on the value of isSuccess
, different variables are assigned and corresponding functions are called.
Conclusion
Mastering the art of writing multiple statements within a ternary operator can significantly enhance your JavaScript coding efficiency. By following the provided guidelines and examples, you can effectively utilize this feature to streamline your code and improve readability.